Count Number of Each Character in Word Document
There may be situations where you want to know how many of each of the letters in the alphabet are found in a Word document, i.e. how many of each of the letters A-Z. Or mayby you want to find out which letters are the most commonly used letters in a specific text.
This article includes two ready-for-use VBA macros that let you get a count of each letter or other character in a Word document.
The macros are made so it is easy for you to include precisely the letters and/or other characters you want. For example, you can add the numbers 0-9 if you want to include the count of each of these digits too.
- MACRO 1 shows the result in a dialog box with the count of each character in the order you specify.
- MACRO 2 shows the result in a dialog box with the character count sorted by the number of occur-rences in descending order, i.e. the character that is found most often is shown first.
Note that the macros count only characters in the main text story. Characters in headers, footers, endnotes, footnotes, etc. are not counted.
Published 1-Mar-2022.
MACRO 1 – Count number of each character in the order you specify
To make it possible for you to adjust the macro to include characters of your own choice, the characters to be checked are specified in a string that you can easily adjust to include the letters or other characters you want.
If you include numbers 0-9 in the string, the occurrence of each of the numbers will also be included.
See the comments in the macro.
You can copy the macro code below and insert it either in your Normal.dotm file or in another Word file of your choice. For help on installing a macro, see How to Install a Macro.
Sub FindNumberOfEachCharacterInActiveDocument_SortedAlphabetically() '========================= 'Macro created 2022 by Lene Fredborg, DocTools - www.thedoctools.com 'THIS MACRO IS COPYRIGHT. YOU ARE WELCOME TO USE THE MACRO BUT YOU MUST KEEP THE LINE ABOVE. 'YOU ARE NOT ALLOWED TO PUBLISH THE MACRO AS YOUR OWN, IN WHOLE OR IN PART. '========================= 'The macro creates a list of the number of occurrences in the active document 'of each character in the list you specify. 'The macro checks the main text story only. 'The result is shown in a dialog box, listed in the character order you specify. '========================= Dim strText As String Dim strTextNew As String Dim lngCount As Long Dim strInfo As String Dim strMsg As String Dim lngTotal As Long Dim strCharacters As String Dim strChar As String 'To make it easy for you to edit the list of characters to count, 'the list is defined as a string, strCharacters. 'Edit the string as desired - for example, add ÆØÅ in case of Danish. '========================= 'The macro does not distinguish between uppercase and lowercase. '========================= strCharacters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" strMsg = "" strText = UCase(ActiveDocument.Range.Text) lngTotal = Len(strText) For lngCount = 1 To Len(strCharacters) 'Get character lngCount from strCharacters strChar = Mid(strCharacters, lngCount, 1) 'To get number of occurrences: 'Replace strChar with "" and calculate difference in length strTextNew = Replace(UCase(strText), strChar, "") strInfo = strChar & ":" & vbTab & lngTotal - Len(strTextNew) & vbCr 'Append info to message strMsg = strMsg & strInfo Next lngCount 'Show information in dialog box strMsg = strMsg & vbCr & vbCr & _ "Total number of characters in main text story: " & lngTotal 'If your list strCharacters is not ordered alphabetially, 'you should edit the MsgBox title below MsgBox strMsg, vbOKOnly, "Character Count Sorted Alphabetically" End Sub
Result of running MACRO 1
Below, you can see an example of the dialog box shown when running MACRO 1 above. The results are sorted alphabetically because the list in strCharacters are sorted that way. The results will be listed in the order you arrange the characters in strCharacters.
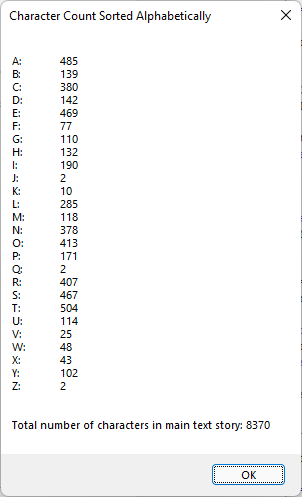
Example of running MACRO 1.
MACRO 2 – Count number of each character in numerical order
This macro is an extension of the macro above. To get the resulting list sorted by the number of occurrences of each character, a temporary Word document is used.
See the comments in the macro.
You can copy the macro code below and insert it either in your Normal.dotm file or in another Word file of your choice. For help on installing a macro, see How to Install a Macro.
Sub FindNumberOfEachCharacterInActiveDocument_SortedByOccurrences() '========================= 'Macro created 2022 by Lene Fredborg, DocTools - www.thedoctools.com 'THIS MACRO IS COPYRIGHT. YOU ARE WELCOME TO USE THE MACRO BUT YOU MUST KEEP THE LINE ABOVE. 'YOU ARE NOT ALLOWED TO PUBLISH THE MACRO AS YOUR OWN, IN WHOLE OR IN PART. '========================= 'The macro creates a list of the number of occurrences in the active document 'of each character in the list you specify. 'The macro checks the main text story only. 'The result is shown in a dialog box, sorted by occurrences of each character in descending order. '========================= Dim strText As String Dim strTextNew As String Dim lngCount As Long Dim strInfo As String Dim strMsg As String Dim lngTotal As Long Dim lngChar As Long Dim strCharacters As String Dim strChar As String Dim oDocTemp As Document Dim oTable As Table Application.ScreenUpdating = False 'To make it easy for you to edit the list of characters to count, 'the list is defined as a string, strCharacters. 'Edit the string as desired - for example, add ÆØÅ in case of Danish. '========================= 'The macro does not distinguish between uppercase and lowercase. '========================= strCharacters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" strMsg = "" strText = UCase(ActiveDocument.Range.Text) lngTotal = Len(strText) 'Create a temporary document to use to store character count and perform sorting 'Prevent AutoNew macro from running, if found WordBasic.DisableAutoMacros 1 Set oDocTemp = Documents.Add 'Insert a table to use for adding information for the final message With oDocTemp .Range.Text = "" 'Insert 2-column table, number of rows = length of strCharacters Set oTable = .Tables.Add(.Range, Len(strCharacters), 2) End With 'Add information about each character in strCharacters For lngCount = 1 To Len(strCharacters) 'Get character lngCount from strCharacters strChar = Mid(strCharacters, lngCount, 1) 'To get number of occurrences: 'Replace strChar with "" and calculate difference in length strTextNew = Replace(UCase(strText), strChar, "") lngChar = lngTotal - Len(strTextNew) 'Insert result in cell 2 in table oTable.Cell(lngCount, 2).Range.Text = lngChar 'Insert character in cell 1 in table oTable.Cell(lngCount, 1).Range.Text = strChar Next lngCount 'Sort table by column 2 and convert to text oTable.Sort ExcludeHeader:=False, FieldNumber:="Column 2", _ SortFieldType:=wdSortFieldNumeric, SortOrder:=wdSortOrderDescending oTable.Rows.ConvertToText Separator:=wdSeparateByTabs 'Store the text from oDocTemp, ready for use in final message strInfo = oDocTemp.Range.Text 'Close oDocTemp without saving oDocTemp.Close wdDoNotSaveChanges Application.ScreenUpdating = True 'Show information in dialog box strMsg = strInfo & vbCr & vbCr & _ "Total number of characters in main text story: " & lngTotal MsgBox strMsg, vbOKOnly, "Character Count Sorted Descending by Number" 'Clean up Set oDocTemp = Nothing Set oTable = Nothing 'Enable auto macros again WordBasic.DisableAutoMacros 0 End Sub
Result of running MACRO 2
Below, you can see an example of the dialog box shown when running MACRO 2 above.
The dialog box shows the character count sorted by the number of occurrences in descending order, i.e. the character that is found most often is shown first.
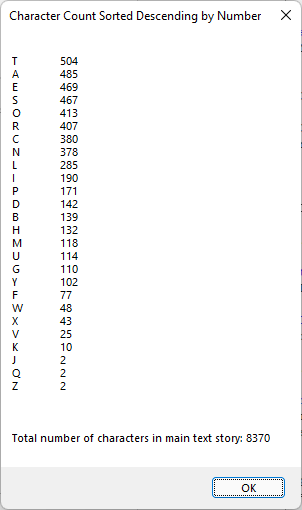
Example of running MACRO 2.
How to changes the macros to count characters in the selection only
Maybe you are interested in counting characters in only part of a document. You can easily change the macros to do so. The following applies to both macros:
In the macro, find the line:
strText = UCase(ActiveDocument.Range.Text)
Change the line to:
strText = UCase(Selection.Text)
Before running the macro, select the text in which you want to count characters.
How to get the total number of characters in a Word document
If you just want to know the total number of characters in a Word document, you can use Word’s built-in Word Count feature.
To open the Word Count dialog box, select the Review tab in the Ribbon > click Word Count.
You can also open the Word Count dialog box via the Status Bar. Right-click in the Status Bar and select Word Count if the option is not already displayed.
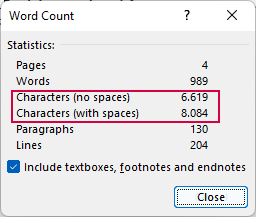
The Word Count dialog box shows the character count both with and without spaces. The dialog box also shows counts of pages, words, paragraphs, and lines.
How to get the total character count using VBA
You can use the VBA code below get the character count in the main text story of a Word document:
ActiveDocument.Characters.Count
Related information
For help on installing a macro, see How to Install a Macro.


Did you know that...
DocTools Word Add-Ins
can help you save time in Word
On my website wordaddins.com you will find some of the Word Add-Ins I have developed, ready for use:
Generate complete documents in seconds from re-usable text or graphics - read more...
helps you manage comments in Word fast and easy – review comments, extract comments, etc. - read more...
Makes it easier than ever to work with cross-references in Word documents - read more...
Lets you manage document data efficiently with custom document properties and DocProperty fields - read more...
Lets you extract insertions, deletions and comments in full context and including headings - read more..
Lets you apply or remove any highlight color by the click of a button - read more...
Browse pages, headings, tables, graphic, etc. and find text in Word with a single click - read more...
Browse pages, headings, tables, graphic, etc. and find text in Word with a single click - read more...
Lets you quickly and easily create screen tips in Word with up to 2040 characters - read more...